Write a program to find the 2’s complements of the number stored at memory location 4400H and stored the complements number at memory location 4300H.
LDA 4400H
CMA
ADI, 01H
STA 4300H
HLT
-> Load the content of memory location 4400 to Accumulator
-> Complement the content of Accumulator
-> Add one to the 1’s Complement (i.e One is added to the content of Accumulator)
-> Store the content of Acculumator to the given memory location of 4300H
WAP in assembly language Program that retrieves data located at 2050H and displays if it is even and stores FFH on that location if it is odd.
LDA 2050H
ANI 01H
JZ Next
MVI A, FFH
STA 2050H
JMP Last
Next: LDA 2050H
OUT 01H
Last: HLT
-> Store the content of memory location 2050 to accumulator
->AND operation with AC and 01, if its AND gives zero then it’s even otherwise it is odd ( A <- A (AND) 01 )
->Jump if ZeroFlag = 1
-> load FFH to AC
-> store the content of AC (which is FF) to the given location 2050H
->Display the content of AC to OUTPUT port 01
Add two numbers located at 3030H and 4040H. Display sum on PORT 1. If carry is generated display it on PORT 2. Store sum on 5050H
LDA 3030H
MOV B, A
LDA 4040H
ADD B
STA 5050H
OUT PORT 1
JNC Last
MVI A, 01H
OUT PORT 2
Last: HLT.
->Load the content which is located at 3030 to Ac
->Move the content to Register B
-> Load accumulator from memory location 4040H
-> Add the content of Ac and B (which are the value of memory locations 4040 and 3030)
-> Store the sum in memory location 5050
-> Display the sum on port 1
-> check to carry if no carry then control will go to the last line otherwise the instruction below it execute
-> immediate the 01 value to Ac
-> Display the carry-on port 2
Write a program to transfer an 8-bit number from 9080H to 9090H if bit D5 is 1 and D3 is 0. Otherwise, transfer data by changing bits D2 and D6 from 1 to 0 or from 0 to 1. Assume there are 10 numbers
LXI H, 9080H
LXI D, 9090H
MVI C, 0AH
L2: MOV A, M
ANI 28H
CPI 20H
JZ L1
MOV A, M
XRI 44H
MOV M, A
L1: MOV A, M
STAX D
INX H
INX D
DCR C
JNZ L2
HLT
-> 00101000=28H( for D5=1 and D3=0 gives 28H)
-> 00100000=20H (If compare is equal to 20 after ANDED with 28H which means at D3 is 0 and D5 is 1)
-> If CPI gives zero results it means D5 is 1
-> 01000100 (Making D2 and D6 bits 1 to 0 or 0 to 1 with X-OR gate)
Write a program for 8085 to change the bit D5 of ten numbers stored at address 7600H if the numbers are larger than or equal to 80H.
LXI H, 7600H
MVI C, 0AH
L2: MOV A, M
CPI 80H
JC L1
XRI 20H
MOV M, A
L1: INX H
DCR C
JNZ L2
HLT
Load the contents 7600 to register pairs HL
Load 10 in register C for the counter.
Move the contents of HL ( M ) into AC.
-> Carry arises if Accumulator content is less than 80H, If carry arises then the number is less than 80H
Jump on carr.
-> 00100000->20H Changing D5 bits using X-OR gate (If the input is the same then its output will be low.)
Move AC content to memory location M (i.e in HL register pair)
Increase the value of HL for the next address to fetch.
Decrease counter by one
Jump on No Zero.
Register BC contained 2793H & register, DE contains 3182H. Write instructions to add these two 16 bits numbers and placed the sum memory location 2050H & 2051H.
MOV A, C
ADD E
MOV L, A
MOV A, B
ADC D
MOV H, A
SHLD 2050H
HLT
Explanation of the above program
-> Load the content of register C to the accumulator
-> Add the content of LSB data of BC i.e C with LSB data of DE i.e E
->Move the addition of two registers C and E which are LSB data if carry is generated then the Cy flag is high.
->Move the content of B to Accumulator and add with D including carry i.e MSB addition
->Move the content of AC to register H.
->SHLD stores the contents of L in a specified location and the contents of H in the next higher location.
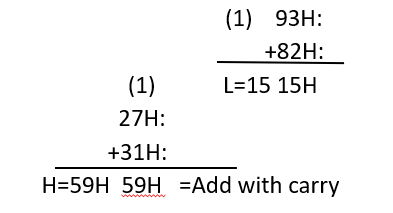
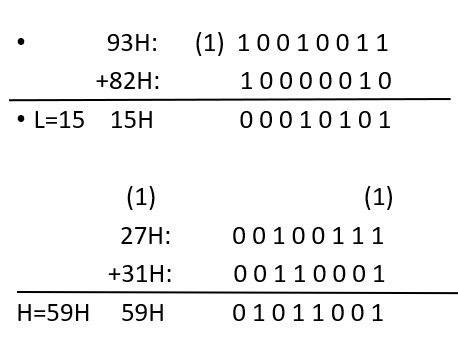
Ten number 8 bits data is stored in the memory location at A000H. WAP for 8085 MP to copy the data to the next table at A030H if the data is less than 70H and greater than 24H
MVI B, 0AH
LXI H, A000H
LXI D, A030H
L2: MOV A, M
CPI 70H
JNC L1
CPI 24H
JC L1
MOV A, M
STAX D
INX D
L1: INX H
DCR B
JNZ L2
HLT
WAP in 8085 MP to transfer 8-bits number from the table to other by setting Bit D5 if the no. is less than 80H otherwise transfer the no. by resetting bit D6
LXI H, 8000H -> SOURCE TABLE
LXI D, 9000H -> DESTINATION TABLE
MVI B, 0AH -> CONSIDER 10 8-BIT NUMBERS
L1: MOV A, M
CPI 80H
JC L2
ANI BFH
JMP L3
L2: ORI 20H ->00100000 = making D5 bits set
L3: STAX D
INX H
INX D
DCR B
JNZ L1
HLT
WAP in assembly language program for 8085 to exchange the bit D6 & D2 of every byte of the program. Supposes there are 200 bytes in the program starting from the memory location 8090H
LXI H, 8090H
MVI B, C8H ->11001000 = 200 byte(200 data)
L1: MOV A, M
ANI 44H ->01000100 = making D6 and D2 1 and other zero to check whether 0 or 1 is present in D6 and D2
MVI C, 08H ->00001000
MVI D, 00H
L2: RLC ->Rotate Accumulator Left without through carry.
JNC L3
INR D
L3: DCR C
JNZ L2
MOV A, D
CPI 01H ->if the content of Ac is 01 then the zero flags become set (1) which means the difference is zero.
MOV A, M
JNZ L4
XRI 44H
L4: MOV M, A
INX H
DCR B
JNZ L1
HLT
A set of 6 data bytes are stored from memory location 2050H. The set includes some blank space (bytes with zero). WAP to remove the blank from the blocks.
MVI C, 06H
LXI H, 2050H
LXI B, 2050H
L2: MOV A, M
CPI 00H
JZ L1
STAX B
INX B
L1: INX H
DCR C
JNZ L2
HLT